初始Mockito
Created At :
Count:441
Views 👀 :
初识Mockito
Mockito是一个针对Java的mocking框架。将模拟对象来代替真实对象。
- 模拟任何外部依赖并将这些模拟对象插入测试代码中
- 执行测试中的代码
- 验证代码是否按照预期执行
前期准备
打开IDEA,new–> project –> Spring Initializr–> ..–>添加Spring Web…–>新建项目。
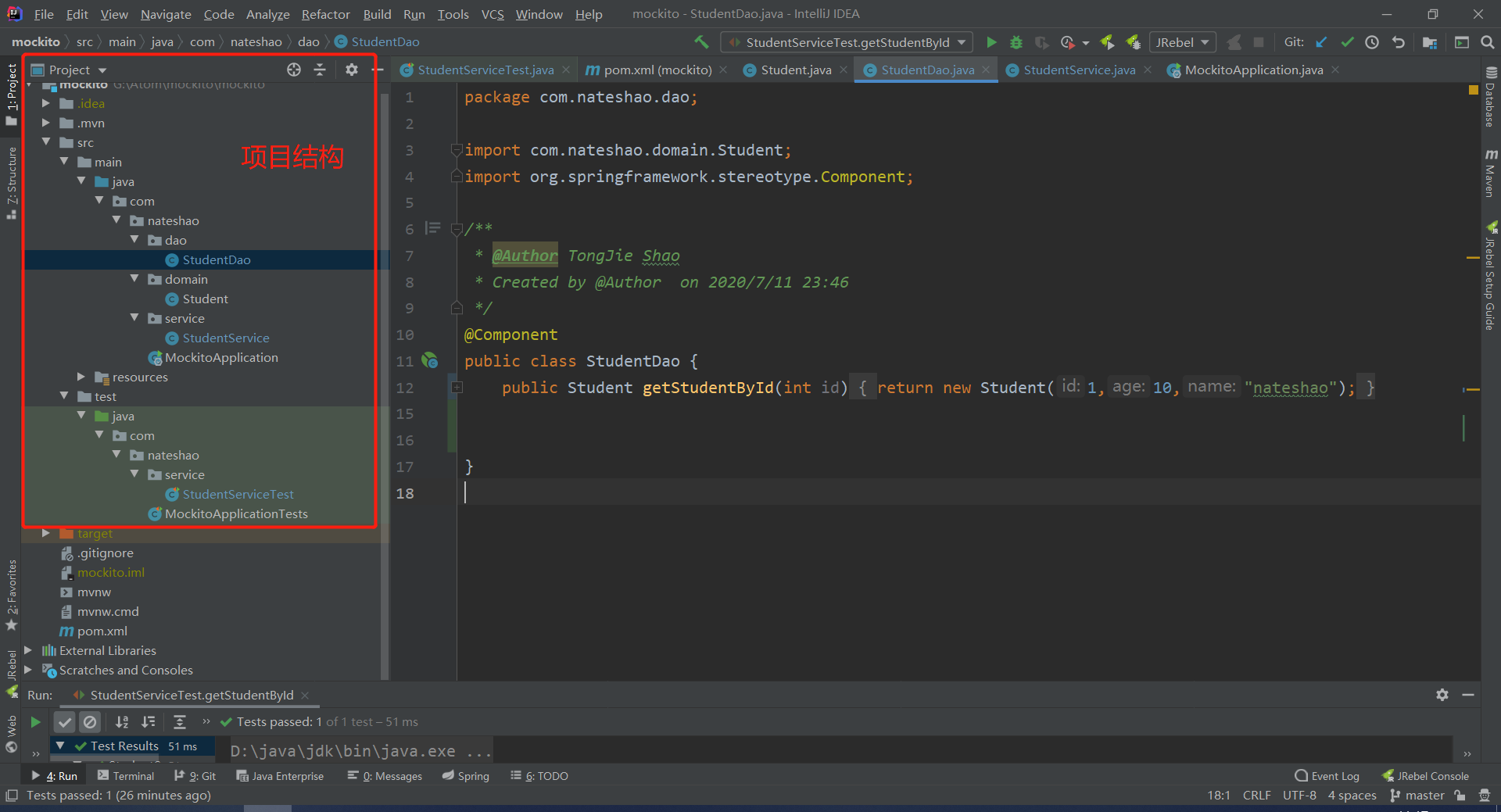
创建Student
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| package com.nateshao.domain;
public class Student { private int id; private int age; private String name;
public Student(int id, int age, String name) { this.id = id; this.age = age; this.name = name; }
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public String getName() { return name; }
public void setName(String name) { this.name = name; } }
|
创建StudentDao
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| package com.nateshao.dao;
import com.nateshao.domain.Student; import org.springframework.stereotype.Component;
@Component public class StudentDao { public Student getStudentById(int id){ return new Student(1,10,"nateshao"); } }
|
创建StudentService
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| package com.nateshao.service;
import com.nateshao.dao.StudentDao; import com.nateshao.domain.Student; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service;
@Service public class StudentService {
@Autowired private StudentDao studentDao;
public Student getStudentById(int id){ return studentDao.getStudentById(id); } }
|
新建测试类StudentServiceTest
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| package com.nateshao.service;
import com.nateshao.dao.StudentDao; import com.nateshao.domain.Student; import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test; import org.mockito.Mockito; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.boot.test.mock.mockito.MockBean;
import static org.junit.jupiter.api.Assertions.*;
@SpringBootTest class StudentServiceTest {
@Autowired private StudentService studentService; @MockBean StudentDao studentDao;
@BeforeEach void setUp() { Mockito.when(studentDao.getStudentById(1)).thenReturn(new Student(1,10,"nateshao")); }
@Test void getStudentById() { Student student = studentService.getStudentById(1); assertNotNull(student); assertEquals(student.getId(),1); assertEquals(student.getAge(),10); assertEquals(student.getName(),"nateshao"); } }
|
测试结果
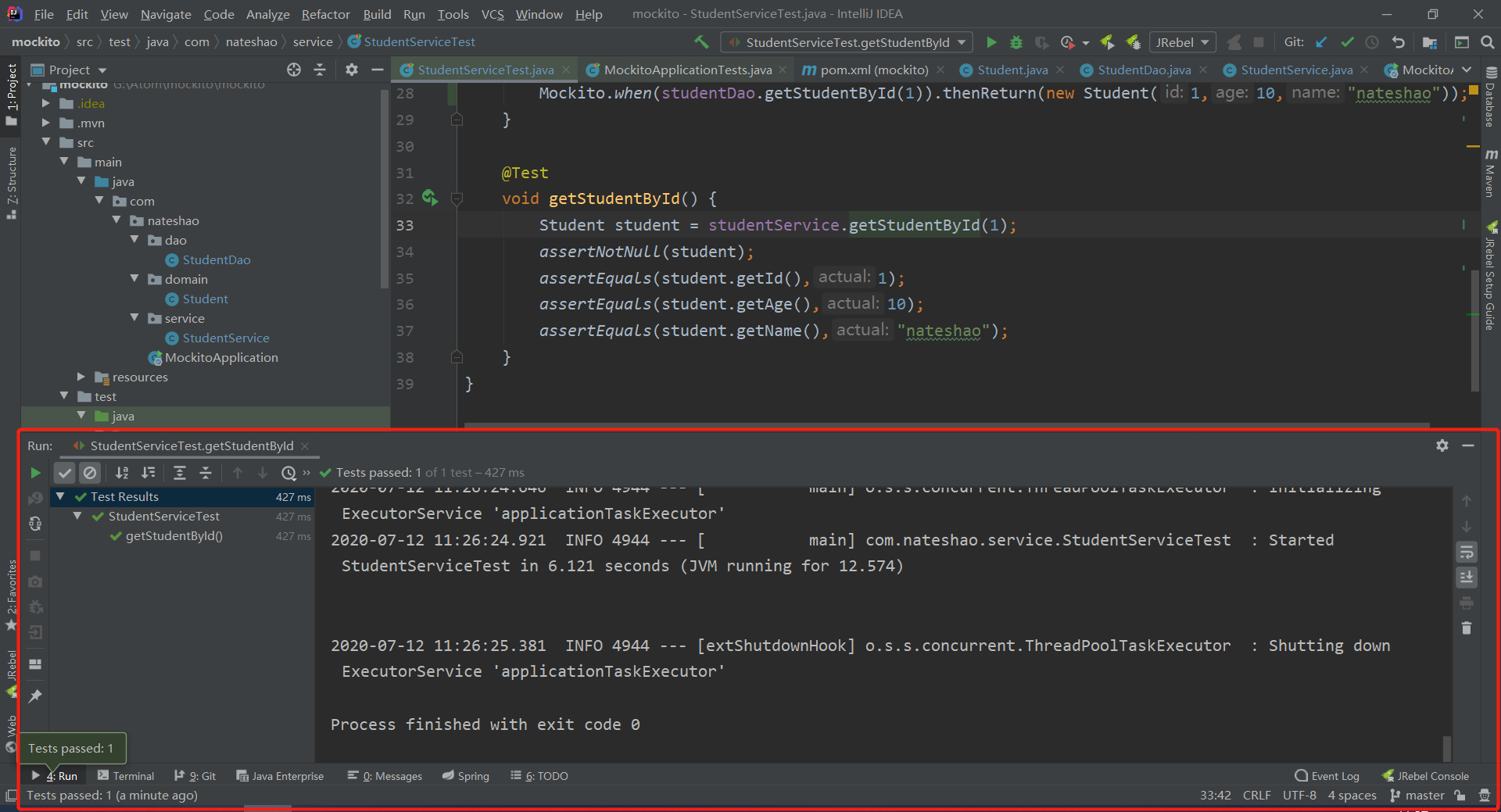
转载请注明来源,欢迎对文章中的引用来源进行考证,欢迎指出任何有错误或不够清晰的表达。可以在下面评论区评论,也可以邮件至 1210331079@qq.com
Title:初始Mockito
Count:441
Author:千 羽
Created At:2020-07-12, 11:32:28
Updated At:2020-08-23, 17:49:48
Url:https://nateshao.github.io/2020/07/12/powermock%E6%B5%8B%E8%AF%95%E7%94%A8%E4%BE%8B/
Copyright: 'Attribution-non-commercial-shared in the same way 4.0' Reprint please keep the original link and author.